Send WhatsApp Messages and validate phone numbers with this PHP script
Automate sending WhatsApp messages, and validate phone numbers using PHP script. Perfect for online stores to notify customers about orders, ensuring messages reach active WhatsApp numbers.
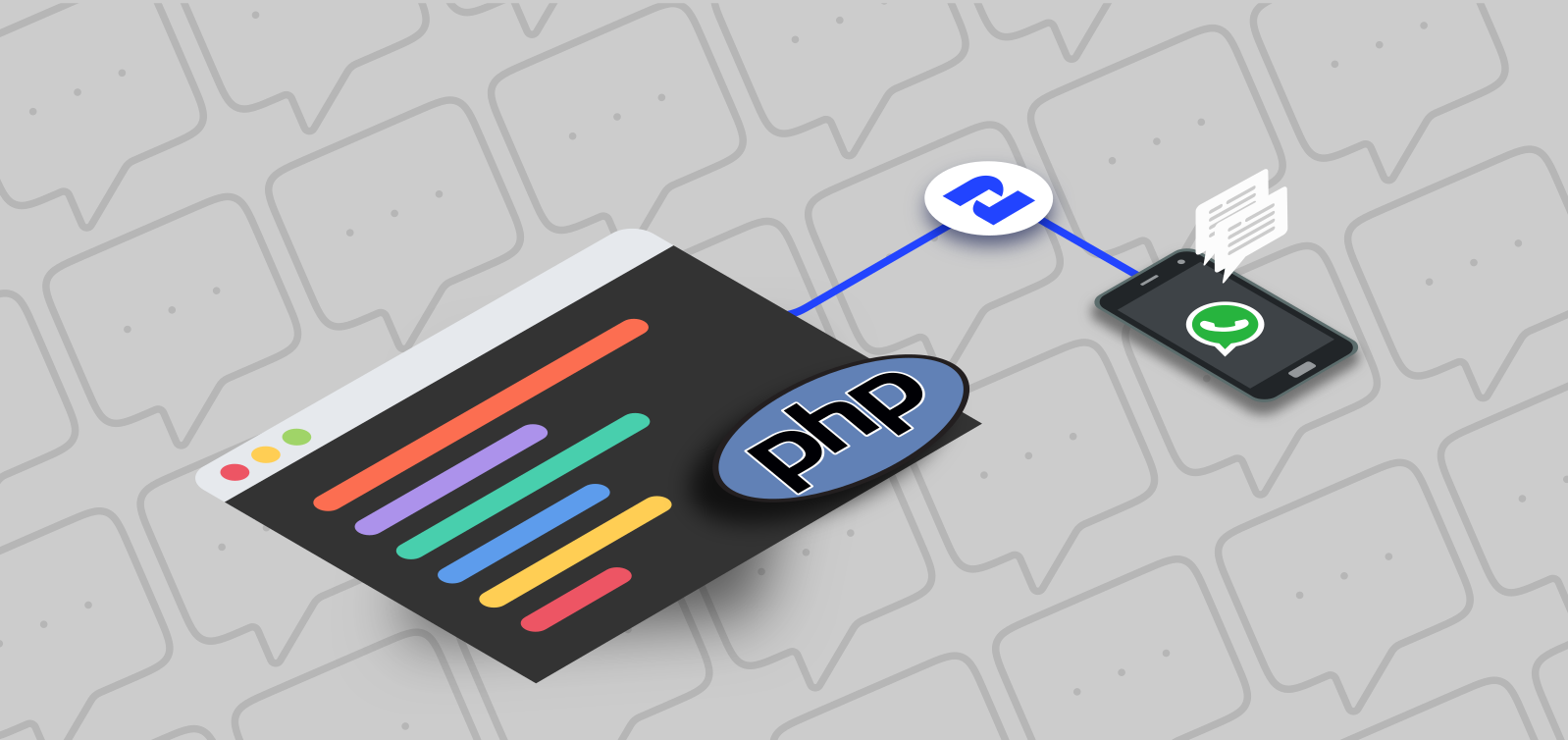
With its massive user base, WhatsApp has become an essential tool for interacting with customers, providing support, and closing sales. But what if you could automate the sending process and validate whether phone numbers are registered on WhatsApp before reaching out?
Imagine you run an online store and need to notify your customers about the status of their orders. With this PHP script, you can send messages automatically and ensure that every phone number provided is active on WhatsApp. This way, you avoid sending messages to inactive numbers, improving the efficiency of your communication and ensuring that important information reaches the right people.
Sending WhatsApp messages and verifying phone numbers with PHP
In this post, we'll show you how to create a PHP script that sends messages directly to WhatsApp and verifies if the recipient's number is registered on the platform. This approach not only optimizes your communication strategy but also saves you time and resources by targeting only active WhatsApp contacts.
Before using this code you need:
- A 2Chat account with a phone number connected
- The API Key that you can get following this article
// Class to interact with the 2Chat API
class TwoChatAPI {
// Variable to store the API key
private $apiKey;
// Base URL for the 2Chat API
private $baseUrl = 'https://api.p.2chat.io';
// Constructor that initializes the API key
public function __construct($apiKey) {
$this->apiKey = $apiKey;
}
/**
* Sends a WhatsApp message using the 2Chat API.
*
* @param string $fromNumber The phone number from which the message is sent.
* @param string $toNumber The phone number to which the message is sent.
* @param string $text The content of the message.
* @param string $url (Optional) URL to be attached to the message.
*
* @return array API response, including the HTTP status code and the response body.
*/
public function sendMessage($fromNumber, $toNumber, $text, $url) {
// Define the endpoint for sending messages
$endpoint = $this->baseUrl . '/open/whatsapp/send-message';
echo("endpoint:".$endpoint);
// Data to be sent in the request
$data = [
'to_number' => $toNumber,
'from_number' => $fromNumber,
'text' => $text,
'url' => $url
];
// Make the API request
return $this->makeRequest($endpoint, $data);
}
/**
* Validates if a phone number is enabled for WhatsApp using the 2Chat API.
*
* @param string $sourcePhone The phone number initiating the validation.
* @param string $destinationPhone The phone number to be validated.
*
* @return array API response, including the HTTP status code and the response body.
*/
public function validatePhoneNumber($sourcePhone, $destinationPhone) {
// Define the endpoint for validating phone numbers
$endpoint = $this->baseUrl . "/open/whatsapp/check-number/$sourcePhone/$destinationPhone";
// Make the API request using the GET method
return $this->makeRequest($endpoint, null, 'GET');
}
/**
* Makes an HTTP request to the 2Chat API.
*
* @param string $endpoint The full URL of the API endpoint.
* @param array|null $data The data to be sent in the request body (for POST requests).
* @param string $method The HTTP method to use (default is 'POST').
*
* @return array API response, including the HTTP status code and the response body.
*/
private function makeRequest($endpoint, $data, $method = 'POST') {
// Initialize cURL
$ch = curl_init($endpoint);
// Set cURL to return the response instead of outputting it directly
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
// Set the HTTP headers
curl_setopt($ch, CURLOPT_HTTPHEADER, [
'Content-Type: application/json',
'X-User-API-Key: ' . $this->apiKey
]);
// If the method is POST, set the request fields
if ($method === 'POST') {
curl_setopt($ch, CURLOPT_POST, true);
curl_setopt($ch, CURLOPT_POSTFIELDS, json_encode($data));
} else {
// If the method is GET, set cURL to perform a GET request
curl_setopt($ch, CURLOPT_HTTPGET, true);
}
// Execute the request and get the response
$response = curl_exec($ch);
// Get the HTTP status code of the response
$httpCode = curl_getinfo($ch, CURLINFO_HTTP_CODE);
// Close the cURL connection
curl_close($ch);
// Return the status code and the decoded response as an array
return [
'status_code' => $httpCode,
'response' => json_decode($response, true)
];
}
}
This PHP script defines a class TwoChatAPI
that allows interaction with the 2Chat API. The class provides two main methods:
sendMessage
: This method sends a WhatsApp message from a specified phone number to another. It takes parameters for the source number enabled as a channel in 2Chat (fromNumber
), destination number (toNumber
), and the text of the message (text
)validatePhoneNumber
: This method checks if a phone number is valid for receiving WhatsApp messages. Validating the source number and the destination number is required.
Both methods use a private method called makeRequest
to handle the HTTP requests to the API. This method manages both POST and GET requests, configuring the cURL connection accordingly and returning the API response along with the HTTP status code.
The class is designed to be user-friendly, with API key authentication handled through the class constructor.
// Usage example:
$apiKey = 'ADD_2CHAT_API_KEY_HERE';
$twoChatAPI = new TwoChatAPI($apiKey);
// Send a message
$result = $twoChatAPI->sendMessage(
'+000000000000', //Replace 000000000000 with then phone number enabled as channel in 2Chat
'+111111111111', // Destination phone number
'Test from 2Chat API',
''
);
// Lets print the result
print_r($result);
// Validate a phone number
$validationResult = $twoChatAPI->validatePhoneNumber(
'+000000000000', //Replace 000000000000 with then phone number enabled as channel in 2Chat
'+111111111111', // Replace 111111111111 with the phone number to validate
);
print_r($validationResult);