Usa PHP para enviar mensajes de WhatsApp y validar números de teléfono
Automatiza el envío de mensajes en WhatsApp y valida números de teléfono con un script en PHP, ideal para tiendas en línea. Notifica a tus clientes sobre sus pedidos y asegúrate de que cada mensaje llegue a números activos, mejorando la eficiencia y evitando contactos inactivos.
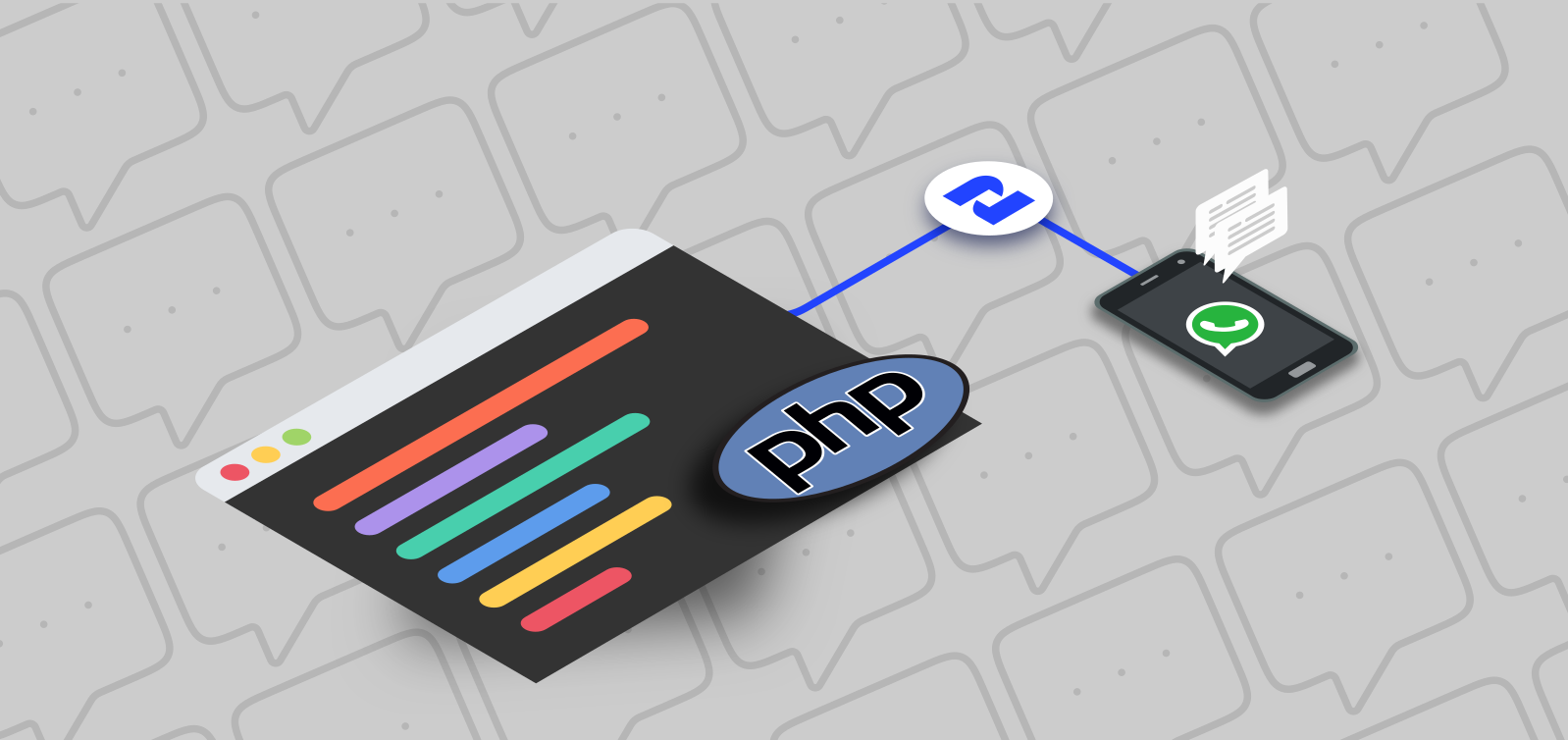
Con su enorme base de usuarios, WhatsApp se ha convertido en una herramienta esencial para interactuar con clientes, ofrecer soporte y cerrar ventas. Pero, ¿y si pudieras automatizar el proceso de envío de mensajes y también validar si los números de teléfono están registrados en WhatsApp antes de contactar?
Imagina que tienes una tienda en línea y necesitas notificar a tus clientes sobre el estado de sus pedidos. Con este script en PHP, no solo puedes enviar mensajes automáticamente, sino también asegurarte de que cada número de teléfono proporcionado esté activo en WhatsApp. De esta manera, evitas enviar mensajes a números inactivos, mejorando la eficiencia de tu comunicación y garantizando que la información importante llegue a las personas correctas.
Envío de mensajes de WhatsApp y verificación de números de teléfono con PHP
En este post, te mostraremos cómo crear un script en PHP que no solo envía mensajes directamente a WhatsApp, sino que también verifica si el número del destinatario está registrado en la plataforma. Este enfoque no solo optimiza tu estrategia de comunicación, sino que también te ahorra tiempo y recursos al dirigirte únicamente a contactos activos en WhatsApp.
Before using this code you need:
- Una cuenta de 2Chat con un número de teléfono conectado.
- La API Key la puedes obtener siguiendo las instrucciones de este artículo.
// Class to interact with the 2Chat API
class TwoChatAPI {
// Variable to store the API key
private $apiKey;
// Base URL for the 2Chat API
private $baseUrl = 'https://api.p.2chat.io';
// Constructor that initializes the API key
public function __construct($apiKey) {
$this->apiKey = $apiKey;
}
/**
* Sends a WhatsApp message using the 2Chat API.
*
* @param string $fromNumber The phone number from which the message is sent.
* @param string $toNumber The phone number to which the message is sent.
* @param string $text The content of the message.
* @param string $url (Optional) URL to be attached to the message.
*
* @return array API response, including the HTTP status code and the response body.
*/
public function sendMessage($fromNumber, $toNumber, $text, $url) {
// Define the endpoint for sending messages
$endpoint = $this->baseUrl . '/open/whatsapp/send-message';
echo("endpoint:".$endpoint);
// Data to be sent in the request
$data = [
'to_number' => $toNumber,
'from_number' => $fromNumber,
'text' => $text,
'url' => $url
];
// Make the API request
return $this->makeRequest($endpoint, $data);
}
/**
* Validates if a phone number is eligible for WhatsApp using the TwoChat API.
*
* @param string $sourcePhone The phone number initiating the validation.
* @param string $destinationPhone The phone number to be validated.
*
* @return array API response, including the HTTP status code and the response body.
*/
public function validatePhoneNumber($sourcePhone, $destinationPhone) {
// Define the endpoint for validating phone numbers
$endpoint = $this->baseUrl . "/open/whatsapp/check-number/$sourcePhone/$destinationPhone";
// Make the API request using the GET method
return $this->makeRequest($endpoint, null, 'GET');
}
/**
* Makes an HTTP request to the 2Chat API.
*
* @param string $endpoint The full URL of the API endpoint.
* @param array|null $data The data to be sent in the request body (for POST requests).
* @param string $method The HTTP method to use (default is 'POST').
*
* @return array API response, including the HTTP status code and the response body.
*/
private function makeRequest($endpoint, $data, $method = 'POST') {
// Initialize cURL
$ch = curl_init($endpoint);
// Set cURL to return the response instead of outputting it directly
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
// Set the HTTP headers
curl_setopt($ch, CURLOPT_HTTPHEADER, [
'Content-Type: application/json',
'X-User-API-Key: ' . $this->apiKey
]);
// If the method is POST, set the request fields
if ($method === 'POST') {
curl_setopt($ch, CURLOPT_POST, true);
curl_setopt($ch, CURLOPT_POSTFIELDS, json_encode($data));
} else {
// If the method is GET, set cURL to perform a GET request
curl_setopt($ch, CURLOPT_HTTPGET, true);
}
// Execute the request and get the response
$response = curl_exec($ch);
// Get the HTTP status code of the response
$httpCode = curl_getinfo($ch, CURLINFO_HTTP_CODE);
// Close the cURL connection
curl_close($ch);
// Return the status code and the decoded response as an array
return [
'status_code' => $httpCode,
'response' => json_decode($response, true)
];
}
}
Explicación del Script
Este script en PHP define una clase llamada TwoChatAPI
que permite interactuar con la API de TwoChat, una plataforma para enviar mensajes a través de WhatsApp. La clase proporciona dos métodos principales:
sendMessage
: Este método permite enviar un mensaje de WhatsApp desde un número especificado a otro número. Recibe como parámetros el número de origen (fromNumber
), el número de destino (toNumber
), el texto del mensaje (text
), y opcionalmente una URL para incluir en el mensaje.validatePhoneNumber
: Este método valida si un número de teléfono es válido para recibir mensajes de WhatsApp. Requiere el número de origen y el número de destino que se quiere validar.
Ambos métodos utilizan un método privado llamado makeRequest
para hacer las solicitudes HTTP a la API. Este método gestiona tanto las solicitudes POST como GET, configurando la conexión cURL según el tipo de solicitud y devolviendo la respuesta de la API junto con el código de estado HTTP.
La clase está diseñada para ser fácil de usar y manejar la autenticación a través de una clave API que se pasa al constructor de la clase.
// Usage example:
$apiKey = 'ADD_2CHAT_API_KEY_HERE';
$twoChatAPI = new TwoChatAPI($apiKey);
// Send a message
$result = $twoChatAPI->sendMessage(
'+000000000000', //Replace 000000000000 with then phone number enabled as channel in 2Chat
'+111111111111', // Destination phone number
'Test from 2Chat API',
''
);
// Lets print the result
print_r($result);
// Validate a phone number
$validationResult = $twoChatAPI->validatePhoneNumber(
'+000000000000', //Replace 000000000000 with then phone number enabled as channel in 2Chat
'+111111111111', // Replace 111111111111 with the phone number to validate
);
print_r($validationResult);